Top Python Interview Questions and Answers
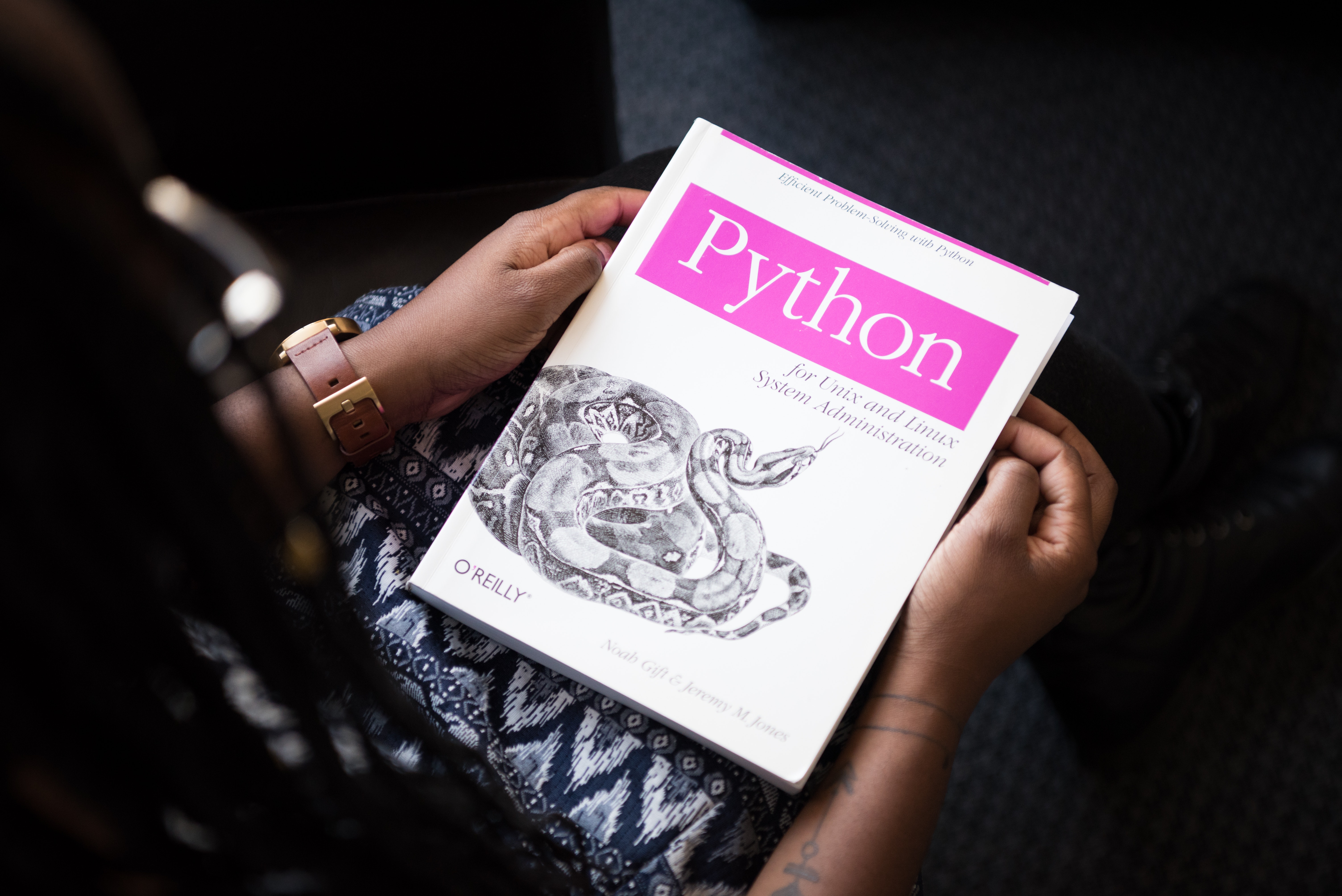
If your dream job is a job in Python, you should be as prepared for the interview bits as possible. Doing some research on the Python interview questions and answers is probably a good idea.
We love Python here at TMS Outsource, so we have composed a list of the Python interview questions you should be familiar to ensure the interview goes well.
So let’s take a look at the top 50 interview questions (and then some) that will help you get ready for a Python interview. From Python interview questions and answers for freshers to the Django interview questions and answers for experienced, we tried to cover it all.
Python Interview Questions
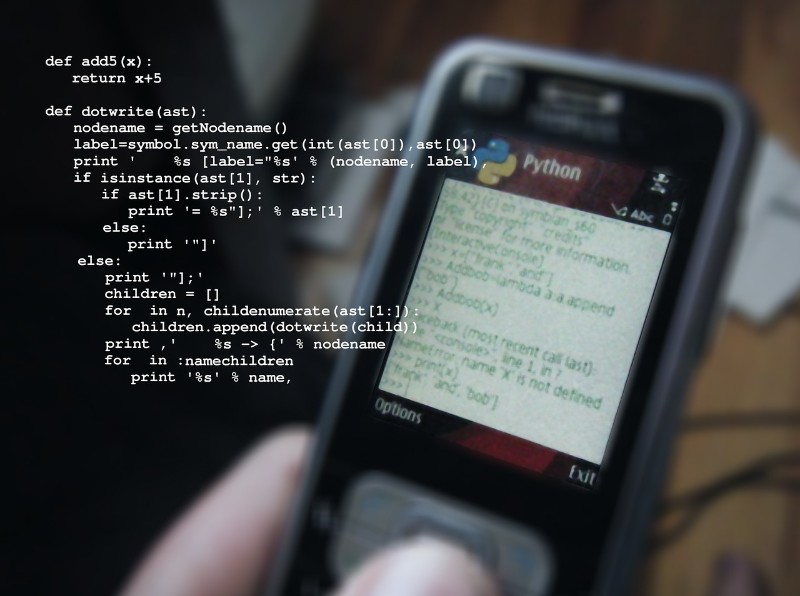
Q. What is Python and can you name some of its features?
A. This is one of the first programming interview questions. You have to know that Python is a programming language. It is object-oriented and interactive and was designed to be highly readable. Unlike other languages, it doesn’t require compilation before running because it is interpreter-based.
There is no need to define the datatypes of the declared variables and it is dynamically typed. For example, you can declare variable x=10 and then x=”Hello World” and it will define the datatype by default depending on its value.
In Python, functions are first-class objects.
This programming language can be used for cross-platform applications including but not limited to big data applications, web applications, scientific models etc.
Q. What is the maximum length of an identifier?
A. This is one of the tricky Python developer interview questions. The truth is that the identifier can be of any length.
Q. What are Decorators?
A. Decorators in Python are used for modifying or injecting code in functions or classes. They are also used to check for permission and for logging the calls.
Q. What is the Memory Management in Python?
A. Python uses a private heap space for its memory management. The data structures, as well as all the objects, are located in a private heap.
Q. Python is one Line. Explain this statement.
A. Python is an interpreted programming language with threads, objects, modules, exceptions etc, while it also has the possibility of automatic memory management.
Q. Explain the interpretation in Python.
A. Programs in python run directly from the source code.
Q. Explain the rules for local and global variables in Python.
A. If the variable is defined outside function then it is global. If, on the other hand, it is an assigned new value inside the function then it is a local variable.
Q. How to share global variable in Python?
A. A global variable can be shared by creating a configuration file store the global variable to be shared across modules.
Q. How to pass optional or keyword parameters from one function to another?
A. We can arrange arguments using the * and ** specifiers in the function’s parameter list.
Q. What are the different types of sequences in Python?
A. Different types of sequences in Python are Strings, Unicode strings, lists, tuples, buffers and, xrange objects.
Q. What is the Lambda form in Python?
A. Lambda keyword is used to create throw-away functions that are small, random, and anonymous.
Q. What is Pickling in Python?
A. Pickle is a standard module which serializes and de-serializes a Python object structure.
Q. How can an object be copied in Python?
A. There are 2 ways to copy objects in Python: Shallow copy & Deep copy.
Q. How do I convert a string to a number?
A. A string can be converted to a number with several different built-functions.
Q. How can you send email from a Python Script?
A. The smtplib module is used to define an SMTP client session object that can be used to send email using Pythons Script.
Q. What is the command used for exiting help command prompt?
A. The command name is “quit”.
Q. Write a sorting algorithm for a numerical dataset in Python.
A. The following code can be used to sort a list in Python:
list = [“1”, “4”, “0”, “6”, “9”]
list = [int(i) for i in list]
list.sort()
print (list)
Q. How will you reverse a list?
A. list.reverse() − Reverses objects on a list.
Q. How will you remove the last object from a list?
A. list.pop(obj=list[-1]) − Removes and returns last object from the list.
Q. What are negative indexes and why are they used?
A. The sequences in Python are indexed. The index consists of both the positive and the negative numbers. The numbers that are positive use ‘0’ as the first index and ‘1’ as the second index.
The index for the negative number starts from ‘-1’ that represents the last index in the sequence and ‘-2’ as the penultimate index. The sequence carries forward like a positive number.
The negative index is used to remove any new-line spaces from the string and allow the string to except the last character that is given as S[:-1]. The negative index is also used to show the index to represent the string in the correct order.
Q. Explain split(), sub(), subn() methods of “re” module in Python.
A. There are 3 methods of Python’s “re” module.
• split() – uses a regex pattern to “split” a given string into a list.
• sub() – finds all substrings where the regex pattern matches and then replace them with a different string
• subn() – it is similar to sub() and also returns the new string along with the no. of replacements.
Q. What is the difference between range & xrange?
A. In terms of functionality, range and xrange are basically the same.
For the most part, xrange and range are the exact same in terms of functionality. They both provide a way to generate a list of integers. The difference, however, is that range returns a Python list object and x range returns an xrange object.
In simpler terms, xrange doesn’t generate a static list at run-time as range does. Instead, it creates the values with a special technique called yielding. With that in mind, if you need to generate a list for a huge range, xrange would probably be the better function to use.
This also applies to those using a memory sensitive system (e.g. mobile phone) because they might experience a Memory Error and their program might crash. This can be a result of range using a lot of memory to create an array of integers.
Q. Explain the map function.
A. The map function executes the function given as the first argument on all the elements of the iterable given as the second argument. If the function given takes in more than 1 arguments, then many iterables are given.
Q. How to get indices of N maximum values in a NumPy array?
A. We can get the indices of N maximum values in a NumPy array using the below code:
import numpy as np
arr = np.array([1, 3, 2, 4, 5])
print(arr.argsort()[-3:][::-1])
Q. What is a Python module?
A. A module in Python allows you to logically organize your code. Basically, it is a script that contains import statements, functions, classes, variable definitions etc. DLL files and zip files can also be modules. You can refer to the module name as a string that is stored in the global variable name.
Q. Name the File-related modules in Python.
A. The Python modules/libraries enable you to manipulate binary files and text files on the file system. With their help, you can easily create new files, copy them, delete them, update their content etc.
The libraries are: os, os.path, and shutil.
os and os.path – modules include functions for accessing the filesystem
shutil – module enables you to copy and delete the files.
Q. Explain the use of “with” statement.
A. The “with” statement in Python is used to open a file, process its data, and close the file without calling a close() method. The “with” statement also provides cleanup activities to make the exception handling simpler.
The general form of with:
with open(“filename”, “mode”) as file-var:
processing statements
note: no need to close the file by calling close() upon file-var.close()
Q. Explain all the file processing modes supported by Python.
A. In Python, there are 3 modes that allow you to process files:
read-only mode, write-only mode, read-write mode, and append mode by specifying the flags “r”, “w”, “rw”, “a” respectively.
A text file can be opened in any one of the above said modes by specifying the option “t” along with
“r”, “w”, “rw”, and “a”, so that the preceding modes become “rt”, “wt”, “rwt”, and “at”.A binary file can be opened in any one of the above said modes by specifying the option “b” along with “r”, “w”, “rw”, and “a” so that the preceding modes become “rb”, “wb”, “rwb”, “ab”.
Q. How many sequence types are supported by Python? What are they?
A. Python supports 7 sequence types. They are str, list, tuple, Unicode, byte array, xrange, and buffer. where xrange is deprecated in python 3.5.X.
Q. How do you perform pattern matching in Python?
A. With the regular Expressions/REs/ regexes, we can specify expressions that match certain elements of the given string. For example, we can define a regular expression to match a single character or a digit, a telephone number, an email address, and similar. The Python’s “re” module provides regular expression patterns and was introduced in the later versions of Python 2.5. With the “re” module, we can search text strings, replace text strings, split text strings based on the defined pattern etc.
Q. How to display the contents of a text file in reverse order?
A.
1. convert the given file into a list.
2. reverse the list by using reversed()
3. Eg: for line in reversed(list(open(“file-name”,”r”))):
4. print(line)
Q. What is the difference between NumPy and SciPy?
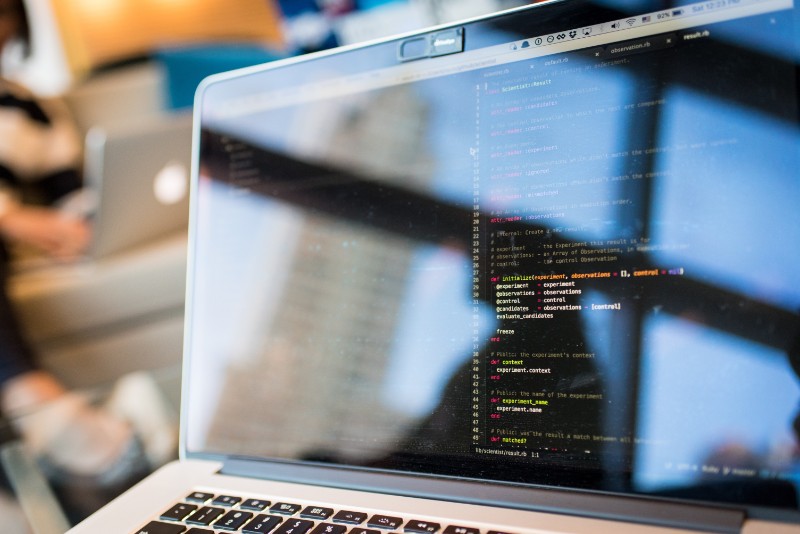
A. There are several ways to describe the difference between NumPy and SciPy when answering Python coding interview questions.
1. In the perfect case, NumPy would contain nothing but the array data type and the most basic operations including indexing, sorting, reshaping, and basic elementwise functions.
2. All numerical code would be contained in SciPy. With that in mind, one of NumPy’s goals is compatibility, so NumPy tries to retain all features supported by either of its predecessors.
3. As a result of that, NumPy contains some linear algebra functions, even though functions of these type actually belong in SciPy. SciPy contains more fully-featured versions of the linear algebra modules, as well as many other numerical algorithms.
4. However, if you are working with Python, you should probably install both NumPy and SciPy. Most new features belong in SciPy rather than NumPy.
Q. Name few Python modules for statistical, numerical, and scientific computations.
A. NumPy – provides an array/matrix type, and it is useful for doing computations on arrays.
Scipy – provides methods for doing numeric integrals, solving differential equations, and similar.
Pylab – a module for generating and saving plots.
Q. What is TkInter?
A. TkInter is a Python library and a toolkit for GUI development. It provides support for various GUI tools or widgets used in GUI apps, including buttons, labels, text boxes, radio buttons, etc. The common attributes of them include fonts, dimensions, cursors, colors etc.
Q. Is Python object-oriented? Explain OOP.
A. This is one of the Python interview questions for freshers and the answer is – yes, Python is object-oriented. Object-Oriented Programming aka OOP is based on classes and instances of those classes called objects. The features of OOP include encapsulation, data abstraction, inheritance, polymorphism etc.
Q. What is multithreading? Give an example.
A. When preparing for Python interview programs, one should know that multithreading means running several different programs at the same time by invoking multiple threads. Threads are light-weight processes communicate with each other to share information. They can be used for a quick task such as calculating results and the can also be running in the background while the main program is running.
Q. Does Python supports interfaces like in Java?
A. When preparing for Python scripting interview questions, one should know the basic differences between Python and other popular programming languages. And one of the differences it the use of the interfaces.
Python doesn’t support interfaces like Java. Abstract Base Class (ABC) and its features are provided by the Python’s ABC module. ABC is a mechanism for specifying the methods that have to be implemented by its implementation subclasses.
Q. What are accessors, mutators, and @property?
A. Accessors and mutators are also known as getters and setters in languages like Java. Python, however, has a @property decorator that allows you to add getters and setters in order to access the attribute of the class.
Q. Explain the difference between append() and extend() methods.
A. Both append() and extend() methods are the methods of the list. They are both used to add the elements at the end of the list.
append(element) – adds the given element at the end of the list
extend(another-list) – adds the elements of another list at the end of the list
Q. Name a few methods that are used to implement Functionally Oriented Programming in Python?
A. One of the things you should know when preparing for the Python programming interview questions is that Python supports methods such as filter(), map(), and reduce().
filter() – enables you to extract a subset of values based on conditional logic.
map() – it is a built-in function that applies the function to each item in an iterable.
reduce() – repeatedly performs a pair-wise reduction on a sequence until a single value is computed.
Q. Explain what Flask is and its benefits?
A. Common Python interview questions often include questions about Python-based frameworks.
You should know that Flask is a microframework that will provide you with the tools, libraries, and technologies for building your own web application. It is very light and comes with only 2 dependencies: Werkzeug a WSGI utility library; jinja2 which is its template engine.
Since Flask is a microframework, there are very few things to update as well as little chances of developing security bugs.
Q. What is the usage of help() and dir() function in Python?
A. Both help() and dir() functions are used for viewing a consolidated dump of built-in functions. They are accessible from Python’s interpreter.
1. Help() function: The help() function is used to display the documentation string while it also allows you to see the help related to modules, keywords, attributes, etc.
2. Dir() function: The dir() function is used to display the defined symbols.
Q. W isn’t all the memory de-allocated in Python?
A. This is one of the Python questions that have multiple answers:
1. With Python and especially with the Python modules having circular references to other objects, the memory is not always de-allocated or freed.
2. It is impossible to de-allocate the portions of the memory reserved by the C library.
3. On exit, because of its own efficient cleanup mechanism, Python tries to de-allocate/destroy every other object.
Q. >>> a = 0101
>>> b = 2
>>> c = a+b
What is the Value of c?
A. When using Python, any number with leading 0 is interpreted as an octal number. So, the variable c will be pointing to the value 67.
Q. How do you represent binary and hexadecimal numbers?
A. If ‘0b’ is leading, Python interprets it as a binary number.
‘0x’ as hexadecimal.
Q. What Arithmetic Operators are supported by Python?
A. ‘+’ : Addition
‘-’ : Subtraction
‘*’ : Multiplication
‘/’ : Division
‘%’ : Modulo division
‘**’ : Power Of
‘//’ : floor div
Python does not support unary operators like ++ or – operators. However, it does support Augmented Assignment Operators;
A += 10 Means A = A+10
B -= 10 Means B = B – 10
Other Operators supported by Python are & , |, ~ , ^ which are and, or, bitwise compliment and bitwise xor operations.
Q. What are the basic Data Types Supported by Python?
A. This is one of the common Python technical interview questions and one should know the following data types:
Numeric Datatypes: int, long, float, NoneType
String: str
Boolean: (True, False)
NoneType: None
Q. How do you check whether the two variables are pointing to the same object in Python?
A. With the so-called ‘is’ operator in Python, you can determine that two variables are pointing to the same object if it returns true.
Example:
>>> a = “Hello world”
>>> c = a
>>> a is c
True
>>> id(a)
46117472
>>> id(c)
46117472
Q. What is for-else and while-else in Python?
A. For-else and while-else refer to Python’s way of handling loops by providing a function to write else block in case the loop is not satisfying the condition.
Example :
a = []
for i in a:
print “in for loop”
else:
print “in else block”
output:
in else block
The same is true with while-else, too.
Q. How do you programmatically know the version of Python you are using?
A. The version property under sys module will give the version of Python that we are using.
>>> import sys
>>> sys.version
‘2.7.12 (v2.7.12:d33e0cf91556, June 27 2016, 15:19:22) [MSC v.1500 32 bit (Intel)]’
>>>
Q. How do you find the number of references pointing to a particular object?
A. The getrefcount() function in the sys module gives the number of references pointing to a particular object including its own reference.
>>> a = “JohnShekar”
>>> b = a
>>> sys.getrefcount(a)
Here, the object ‘JohnShekar’ is referred by a, b and getrefcount() function itself. So the output is 3.
Q. How do you dispose of a variable in Python?
A. ‘del’ is the keyword statement used in Python to delete a reference variable to an object.
>>> a = ‘Hello world!!’
>>> b = a
>>> sys.getrefcount(a)
3
>>> del a
>>> sys.getrefcount(a)
Traceback (most recent call last):
File “<pyshell#23>”, line 1, in <module>
sys.getrefcount(a)
NameError: name ‘a’ is not defined
>>> sys.getrefcount(b)
2
Q. Write a program to check whether a given number is a prime number?
Synopsys:
———————-
Python checkprime.py
Enter Number:23
23 is a prime number
Python checkprime.py
Enter Number:33
33 is a not prime number
A. Solution:
A = input(“Enter Number:”)
for j in xrange(2, int(A**0.5)+1):
if a%j == 0:
print A, ‘Not a prime number’
break
else:
print A, ‘This is a prime number’
Q. What is the difference between range() and xrange() functions in Python?
A. range() is a function that returns a list of numbers and it will be an overhead if the number is too big. xrange(), on the other hand, is a generator function that returns an iterator which returns a single generated value whenever it is called.
Q. What are the generator functions in Python?
A. A generator function is any function that contains at least one yield statement. The difference between yield and return is what makes all the difference here. A return statement terminates the function, while the yield statement pauses, saves all states, and later continues from there on successive calls.
Q. Write a generator function to generate a Fibonacci series?
A. Solution:
def fibo(Num):
a, b = 0, 1
for I in xrange(Num):
yield a,
a, b = b, a+b
Num = input(‘Enter a number:’)
for i in fibo(Num):
print i
Q. What are the ideal naming conventions in Python?
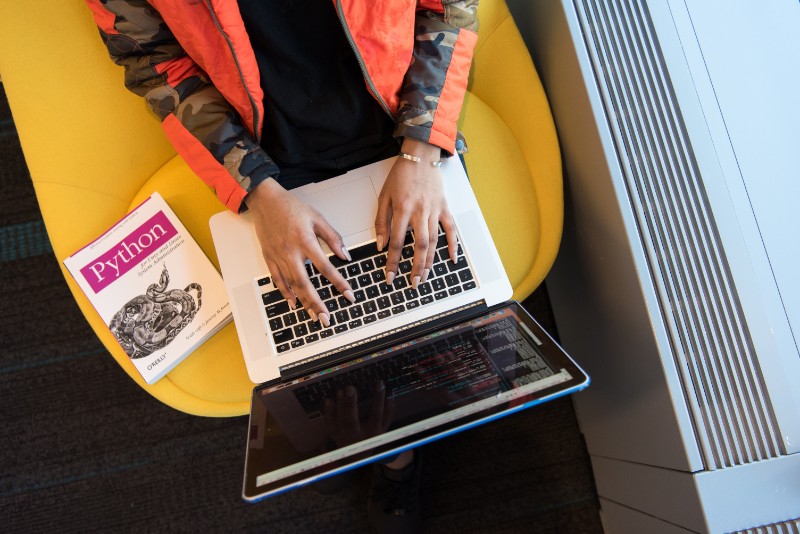
A. The ideal naming conventions in Python are lowercase and underscore.
Examples: is_prime(), test_var = 10 etc
Constants are all either uppercase or camel case.
Example: MAX_VAL = 50, PI = 3.14
None, True, False are predefined constants follow camel case, etc.
Class names are also treated as constants and follow camel case.
Example: UserNames
Q. What happens in the background when you run a Python file?
A. All interview questions aren’t always about what happens in the first plan. One should also be well-aware what happens in the background when you run a .py file.
When you run a Python file, it undergoes two phases. In the first phase, it checks the syntax. In the second phase, it compiles to bytecode (.pyc file is generated) using the Python virtual machine, loads the bytecode into memory, and runs.
Q. What is a module in Python?
A. A module is a Python file where variables, functions, and classes can be defined. It can also have a runnable code and you will recognize it by the .py extension.
Q. How do you create a list which is a reverse version on another list in Python?
A. Python provides a function called reversed(). Here is an example of how it should be used:
>>> a.format(name=’john’, age=’18’)
‘Name: john, age: 18’
>>> a =[10,20,30,40,50]
>>> b = list(reversed(a))
>>> b
[50, 40, 30, 20, 10]
>>> a
[10, 20, 30, 40, 50]
Q. What is a dictionary in Python?
A. Dictionaries in Python are in a way similar to the traditional dictionaries. Their purpose is to serve as a map in another language and they consist of keys and values. Keys are unique, and values are accessed using keys. Here are a few examples of creating and accessing dictionaries.
Examples:
>>> a = dict()
>>> a[‘key1’] = 2
>>> a[‘key2’] = 3
>>> a
{‘key2’: 3, ‘key1’: 2}
>>> a.keys() # keys() returns a list of keys in the dictionary
[‘key2’, ‘key1’]
>>> a.values() # values() returns a list of values in the dictionary
[3, 2]
>>> for i in a: # Shows one way to iterate over a dictionary items.
print i, a[i]
key2 3
key1 2
>>> print ‘key1’ in a # Checking if a key exists
True
>>> del a[‘key1’] # Deleting a key value pair
>>> a
{‘key2’: 3}
Q. How do you merge one dictionary with another?
A. Python provides an update() method which can be used to merge one dictionary with the others.
Example:
>>> a = {‘a’:1}
>>> b = {‘b’:2}
>>> a.update(b)
>>> a
{‘a’: 1, ‘b’: 2}
Q. How to walk through a list in a sorted order without sorting the actual list?
A. This can easily be done in Python with the use of the sorted() function. This function returns a sorted list without modifying the original one. Here is the code:
>>> a
[3, 6, 2, 1, 0, 8, 7, 4, 5, 9]
>>> for i in sorted(a):
print i,
0 1 2 3 4 5 6 7 8 9
>>> a
[3, 6, 2, 1, 0, 8, 7, 4, 5, 9]
>>>
Q. names = [‘john’, ‘fan’, ‘sam’, ‘megha’, ‘popoye’, ’tom’, ‘jane’, ‘james’,’tony’]
Write one line of code to get a list of names that start with character ‘j’?
- Solution:
>>> names = [‘john’, ‘fan’, ‘sam’, ‘megha’, ‘popoye’, ‘tom’, ‘jane’, ‘james’, ‘tony’]
>>> jnames=[name for name in names if name[0] == ‘j’] #One line code to filter names that start with ‘j’
>>> jnames
[‘john’, ‘jane’, ‘james’]
>>>
Q. a = [0, 1, 2, 3, 4, 5, 6, 7, 8, 9]
Write a generator expression to get the numbers that are divisible by 2?
- Solution:
>>> a = [0, 1, 2, 3, 4, 5, 6, 7, 8, 9]
>>> b = (i for i in a if i%2 == 0) #Generator expression.
>>> for i in b: print i,
0 2 4 6 8
>>>
Q. What is a set?
A. A Set is an unordered collection of unique objects.
Q. What is *args and **kwargs?
A. *args is used when we aren’t sure how many arguments are going to be passed to a function. It is also used when the programmer is expecting a list or a tuple as an argument to the function.
**kwargs is used when a dictionary (keyword arguments) is expected as an argument to the function.
Q. >>> def welcome(name=’guest’,city): print ‘Hello’, name, ‘Welcome to’, city
What happens with the following function definition?
A. If you want to pass Python interview coding questions, you have to be able to recognize syntax errors in the code – such as this one. The issue here starts with the function definition. The default argument is following the non-default argument.
According to Python function definition rules, that is not allowed and it leads to an error which causes the program not to run properly or not to run at all. Non-default arguments should be placed first, followed by the default arguments in the function definition. Here is the right way of defining:
def welcome(city, name=’guest’): print ‘Hello’, name, ‘Welcome to’, city
The order of passing values to a function is, first one has to pass non-default arguments, default arguments, variable arguments, and keyword arguments.
Q. Name some standard Python errors.
A. TypeError: Occurs when the expected type doesn’t match with the given type of a variable.
ValueError: When an expected value is not given- if you are expecting 4 elements in a list and you gave 2.
NameError: When trying to access a variable or a function that is not defined.
IOError: When trying to access a file that does not exist.
IndexError: Accessing an invalid index of a sequence will throw an IndexError.
KeyError: When an invalid key is used to access a value in the dictionary.
We can use dir(__builtin__) will list all the errors in Python.
Django Interview Questions
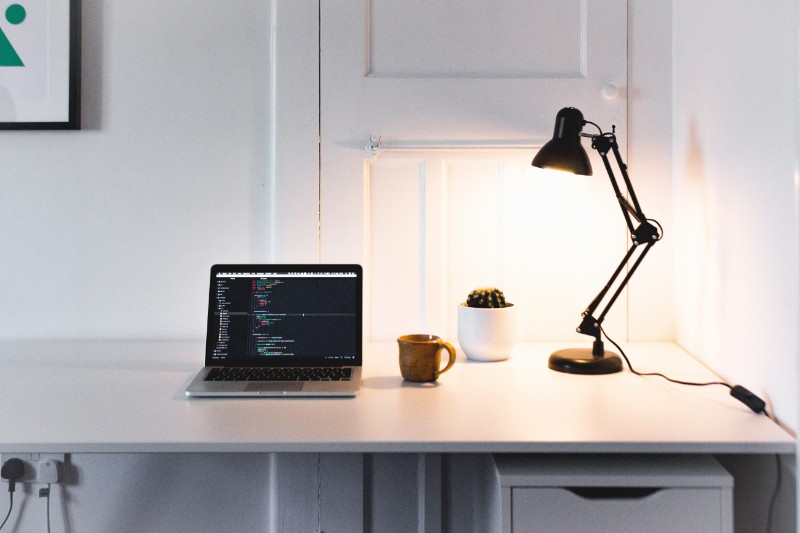
Q. Explain how you can set up the Database in Django.
A. Setting up a Database in Django is quite easy. You can use the regular Python module with a module level representing Django settings. The command you need is edit mysite/setting.py
Django uses SQLite by default. However, if your database choice is different, you will have to change databases by matching your database connection settings with the default database.
- Engines: you can change database by using ‘django.db.backends.sqlite3’ , ‘django.db.backeneds.mysql’, ‘django.db.backends.postgresql_psycopg2’, ‘django.db.backends.oracle’ and so on
- Name: The name of your database. In the case you are using SQLite as your database, it will be a file on your computer. Name should be a full absolute path, including the file name of that file.
- If you are not choosing SQLite as your database, settings like Password, Host, User, etc. must be added.
Since Django’s default database is SQLite, if you have a database server that you want to use instead (for example, PostgreSQL, MySQL, Oracle, MSSQL etc.), you need to create a new database for your Django project with the database’s administration tools. One way or the other, with your database in place, you have to tell Django how to use it. This is where your project’s settings.py file comes in.
Q. What do the Django templates consist of?
A. Every template is basically just a simple text file and it can be created in all kinds of text-based formats such as CSV, HTML, XML, and others. A template consists of variables that are replaced with values when the template is evaluated and of tags (% tag %) that control the logic of the template.
Q. Explain the use of session in the Django framework.
A. The session in the Django framework lets you store and retrieve data on a per-site-visitor basis. By placing a session ID cookie on the client side and storing all the related data on the server side, Django abstracts the process of sending and receiving cookies.
Q. List out the inheritance styles in Django.
A. There are three possible inheritance styles in Django:
1. Abstract Base Classes – used when you only want parent’s class to hold information that you don’t want to type out for each child model.
2. Multi-table Inheritance – used when you are sub-classing an existing model and need each model to have its own database table.
3. Proxy models – used when only want to modify the Python level behavior of the model without changing the model’s fields.
Q. What advantages do NumPy arrays offer over (nested) Python lists?
A. This is one of the Python advanced interview questions and there are multiple things to consider when answering it.
1. Python’s lists are efficient general-purpose containers and they support insertion, deletion, appending, and concatenation. Python’s list comprehensions make them easy to construct and manipulate.
2. There are certain limitations. For example, they don’t support vector-based operations like elementwise addition and multiplication. In addition to that, they can contain different types of objects. In a case like that, Python has to store type information for every element and execute type dispatching code when operating on each element.
3. NumPy is not just more convenient as well as more efficient. It comes with a variety of free vector and matrix operations which help you not to deal with a lot of extra work. In addition to that, they are also efficiently implemented.
4. NumPy array is faster and it comes with built-in FFTs, convolutions, fast searching, basic statistics, linear algebra, histograms, etc.
Q. Explain the use of decorators.
A. Decorators are used for modifying or injecting code in functions or classes. For example, they enable you to wrap a function or a class method call in order for that piece of code to be executed before or after the execution of the original code.
They are also often used for checking for permissions, logging the calls to a specific method, modifying or tracking the arguments passed to a method etc.
Q. How do you make 3D plots/visualizations using NumPy/SciPy?
A. Keep in mind that 3D graphics is beyond the scope of NumPy and SciPy. However, just like in the case of 2D, there are several packages available that can integrate with NumPy for basic 3D plotting. For example, Matplotlib provides basic 3D plotting in the mplot3d subpackage. Mayavi, on the other hand, provides a wide range of high-quality 3D visualization features, utilizing the powerful VTK engine.
Ending thoughts on Python interview questions
There is a lot to know about Python when getting ready for an interview. Hopefully, these basic Python interview questions can help you to be as prepared as possible. We covered all the common interview questions on Python, some more detailed Python data science interview questions, as well as the basic Python coding interview questions and answers.
All there is left to say is – Good luck!
If you enjoyed reading this article on Python interview questions, you should check out this one about Python vs PHP.
We also wrote about a few related subjects like web developer interview questions, Node.js interview questions, React interview questions, best IDE for web development, Python web development and Python frameworks.